Git Cheat Sheet


This post contains affiliate links. This means at no extra cost to you, I may earn a commission if you purchase through my links. Please see my website disclaimer for more info.
What is Git?
In a nutshell, Git is a free and open source distributed version control system designed to handle everything from small to very large projects with speed and efficiency.
What is Version Control?
Version control is a system that records changes to a file, or set of files over time so that you can recall, modify, and revert specific versions later.
How does Git differ from other version control systems?
Git is a distributed version control system, which means that the entire codebase and history is available on every developer’s computer, which allows for easy branching and merging.
Common Git Commands
Below is a list of some common Git commands that you might find useful. I’ve tried to keep the list as short as possible, but still include the most common commands that you’ll need for most projects.
Quick Nav
- Configuring Git
- Configuring a Repository
- Making Local Changes
- Inspecting a Repository
- Working with Remotes
- Branching
- Merging & Rebasing
- Stashing
- Tagging
Configuring Git
Set Your Commit Name and Email
Example
git config [--global] user.name "Your Name"
git config [--global] user.email "Your Email"
Explanation
Configures the username and email used for commits for the current repository, or optionally, globally for all repositories if the --global
flag is used.
List Configuration Settings
Example
git config --list
Explanation
Lists all Git configuration settings, showing both global and local configurations.
Configuring a Repository
Initialize a Repository
Example
git init
Explanation
Initializes a new Git repository in the current directory, creating a .git
subdirectory to store version control information.
Note: You can use
git init <directory>
to initialize a repository in a specific directory.
Set the Default Branch Name
Example
git config --global init.defaultBranch <branch_name>
Explanation
Sets the name of the main branch to be created when you initialize a new repository. This is useful if you want to use a branch name other than master
.
Note: If you just want to change the name of the main branch in an existing repository, you can use
git branch -m <branch_name>
.
Clone a Repository
Example
git clone <repository_url> [local_directory]
Explanation
Creates a local copy of a remote repository, including all branches and commit history. Using git clone
automatically sets up a remote connection called origin
pointing back to the cloned repository.
Optionally, you can specify a directory name to clone into, which will create a new directory with that name to clone into. If you don’t specify a directory name, Git will use the repository name as the directory name.
Note: Using
git clone
will download all code, but also the entire git history of that repository. If you want to clone a repository without the history, you can use degit, just make sure you verify the license of the code you’re cloning to verify that you’re allowed to do so.
Making Local Changes
Adding Files
Example
git add <file>
Explanation
Stages changes for commit. You can specify individual files or use git add .
to stage all changes in the current directory.
Unstaging Added Files
Example
git restore --staged <file>
Explanation
Unstages a file, removing the changes from the staging area. This does not discard the changes from the working directory, it just removes them from the staging area.
Moving Files
Example
git mv <old_file> <new_file>
Explanation
Renames or moves a file and stages the change for the next commit.
Removing Files
Example
git rm <file>
Explanation
Removes a file from both your working directory and the version control. It stages the removal for the next commit.
Discarding Local Changes
Example
git restore <file>
Explanation
Discards local changes in a specific file. This command is similar to git checkout
, but safer, as it will not discard uncommitted changes.
Committing Local Changes
Example
git commit -m "Commit message"
Explanation
Creates a new commit with the staged changes along with a descriptive message. This will only commit changes that have been staged with git add
, and will only commit changes locally.
Adding to the Most Recent Commit
Example
git commit --amend
Explanation
Rewrites the most recent commit with the staged changes and/or a new commit message. Useful if you’ve already pushed the commit to a remote repository, but need to add more changes or fix a typo in the commit message.
Undoing Local Commits
Example
git reset <commit_hash>
Explanation
Undoes all commits after <commit_hash>
, preserving changes locally.
Note: You can use
git reset --hard <commit_hash>
to discard all changes after<commit_hash>
.
Inspecting a Repository
Viewing the Repository Status
Example
git status
Explanation
Displays the status of your working directory, showing which files are modified, staged, or untracked.
Viewing the Commit History
Example
git log [--oneline]
Explanation
Displays the commit history, starting with the most recent commit. By default, it shows the commit ID, author, date, and commit message for each commit. You can use git log --oneline
to show a simplified log with each commit’s hash and subject on a single line.
Viewing Changes
Example
git diff [file]
Explanation
Shows differences between your working directory and the last committed version. Optionally, if you specify a file, it will only show the differences for that file.
Viewing a Specific Commit
Example
git show <commit_hash>[:<file>]
Explanation
Shows the details of a specific commit, including the changes made and the commit message. Optionally, if you follow the commit hash by a colon (:) and a file name, it will only show the changes for that file.
Viewing Who Made Changes to a File
Example
git blame <file>
Explanation
Displays line-by-line annotations, showing which commit and author last modified each line of a file.
Working with Remotes
Adding a Remote Repository
Example
git remote add <name> <repository_url>
Explanation
Adds a new remote repository on your local machine. The name is often origin
, but can be anything you want. The URL is the location of the remote repository.
Note: You need to first use
git init
orgit clone
before you can add a remote repository.
Viewing Remote Repositories
Example
git remote -v
Explanation
Displays the URLs of remote repositories associated with your local repository.
Renaming a Remote Repository
Example
git remote rename <old_name> <new_name>
Explanation
Renames a remote repository. This is useful if you want to rename the origin
remote to something else.
Removing a Remote Repository
Example
git remote remove <name>
Explanation
Removes a remote repository from your local machine.
Fetching Changes
Example
git fetch origin
Explanation
Retrieves changes from a remote repository, but doesn’t automatically merge or apply them. Useful for reviewing changes before merging.
Pushing Changes
Example
git push origin <branch>
Explanation
Uploads your local commits to a remote repository, updating the remote branch.
Pulling Changes
Example
git pull origin <branch>
Explanation
Fetches changes from a remote repository and merges them into your current branch.
Note 1: You can use
git pull --rebase
to fetch changes and rebase your local commits on top of them.
Note 2: To discard any local changes and overwrite them with the remote changes, use
git fetch origin && git reset --hard origin/<branch>
.
Syncing Filename Changes to Remote Repository
Example
git rm -r --cached .
git add --all .
Explanation
Fixes filename case mismatches between your local and remote repository if/when git cached the old name(s). This is useful if you’re having issues with filename case mismatches between your local and remote repositories.
Undoing Public Commits
Example
git revert <commit_hash>
Explanation
Undoes a public commit, creating a new commit with the inverse of the changes. This is useful if you’ve already pushed the commit to a remote repository.
Branching
Creating a Branch
Example
git branch <branch>
Explanation
Creates a new branch. This does not switch to the new branch, it just creates it.
Note 1: You can use
git checkout -b <branch>
to create a new branch and switch to it.
Note 2: If you omit the branch name, it will list all local branches.
Viewing all Branches
Example
git branch
Explanation
Lists all local branches. The current branch is highlighted with an asterisk (*).
Switching Branches
Example
git checkout <branch>
Explanation
Switches to a different branch. Can also be used to create and switch to a new branch (git checkout -b new-branch
).
Deleting Branches
Example
git branch -d <branch>
Explanation
Deletes a branch. This is a “safe” delete, as it will not delete the branch if it has unmerged changes.
Note: You can use
git branch -D <branch>
to force delete a branch, even if it has unmerged changes.
Pruning Remote Branches
Example
git remote prune origin
Explanation
Removes references to remote branches that have been deleted on the remote repository.
Merging & Rebasing
Merging Changes
Example
git merge <branch>
Explanation
Integrates changes from one branch into the current branch. Used to combine work from different branches.
Aborting a Merge
Example
git merge --abort
Explanation
Aborts a merge, reverting back to the state before the merge began.
Rebasing
Example
git rebase <branch>
Explanation
Applies commits from one branch onto another, often used to integrate changes and maintain a linear commit history. This is useful if you started a branch, but another branch has been merged into the main branch, and you want to update your branch with the latest changes.
Stashing
Stashing Changes
Example
git stash save "Work in progress"
Explanation
Temporarily saves changes not ready for commit. Useful for switching branches without committing incomplete work.
Viewing Stashes
Example
git stash list
Explanation
Lists all stashes, showing the branch and message for each stash.
Applying Stashes
Example
git stash apply
Explanation
Applies the most recent stash to the current branch, leaving the stash intact. Or in simpler terms, it restores the changes from the stash.
Deleting Stashes
Example
git stash drop
Explanation
Deletes the most recent stash.
Tagging
Creating a Tag
Example
git tag <tag_name>
Explanation
Creates a named tag at the current commit, often used to mark release versions.
Viewing Tags
Example
git tag
Explanation
Lists all tags in alphabetical order.
Pushing Tags
Example
git push origin <tag_name>
Explanation
Uploads the specified tag to a remote repository.
Deleting Tags
Example
git tag -d <tag_name>
Explanation
Deletes a tag locally.
Checking Out Tags
Example
git checkout <tag_name>
Explanation
Checks out the specified tag, putting you in a “detached HEAD” state, where you can look around and make experimental changes, but you can’t commit. Or in simpler terms, it switches to the specified tag.
Well damn, that was quite the list! It’s probably more than you’ll need for most projects, but it’s good to have as a reference when you need it and should cover most of your needs.
Until next time,
michael 😀
Share this post:

Google Apps Script: 2 Caching Methods You Need to Use!
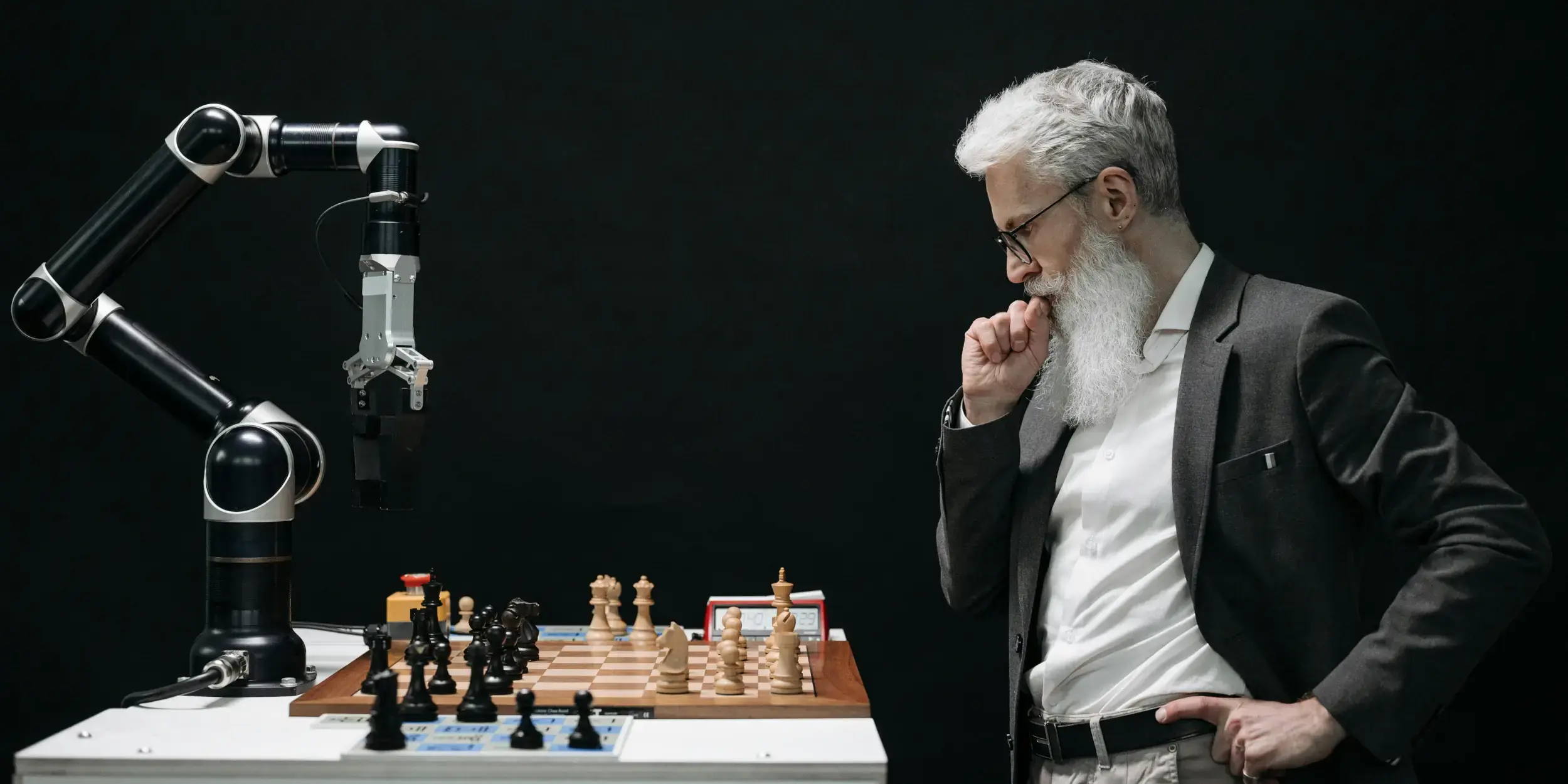
In a World with AI, Where Will the Future of Developers Lie?
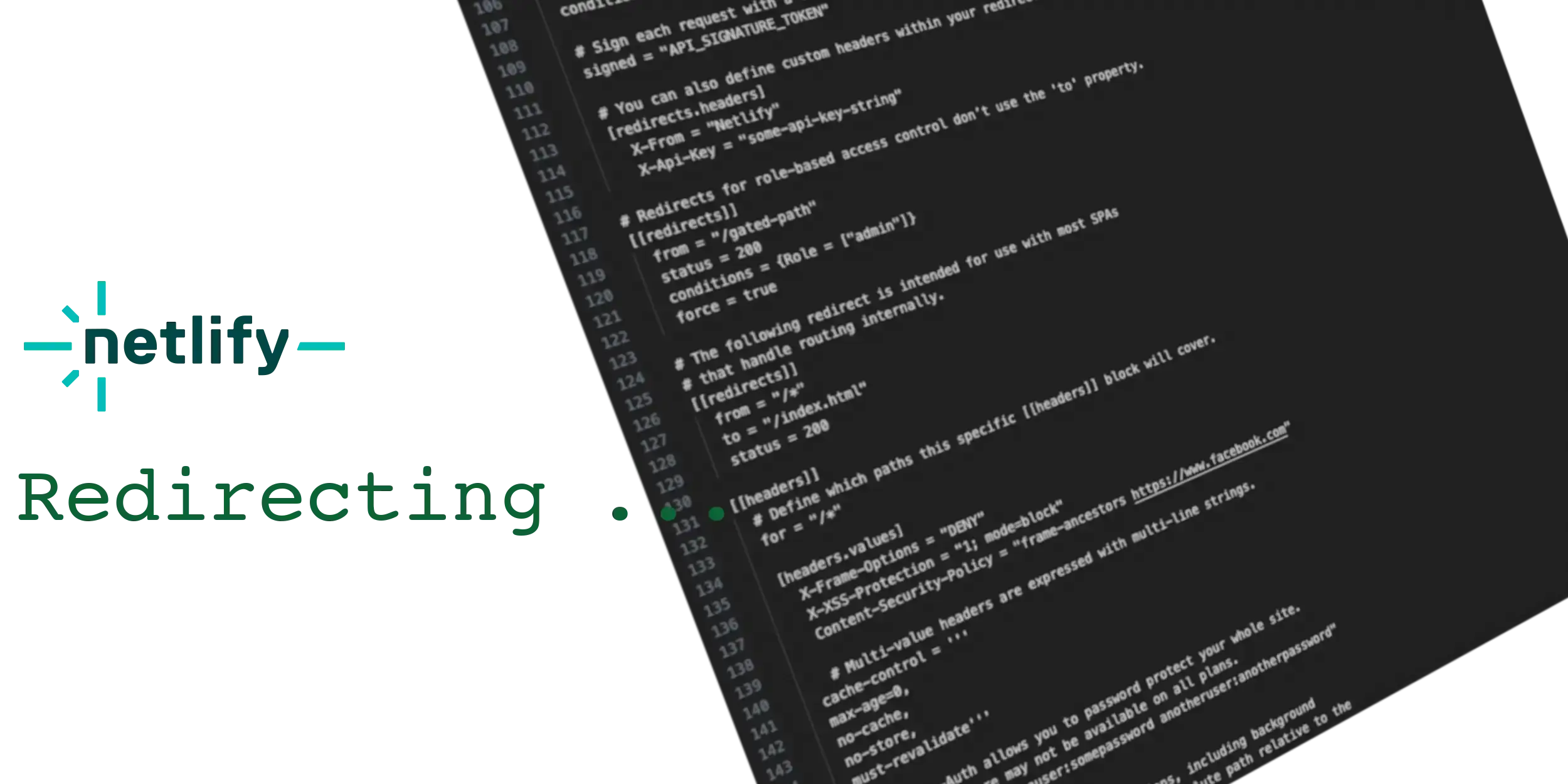
How to Redirect URLs with Netlify
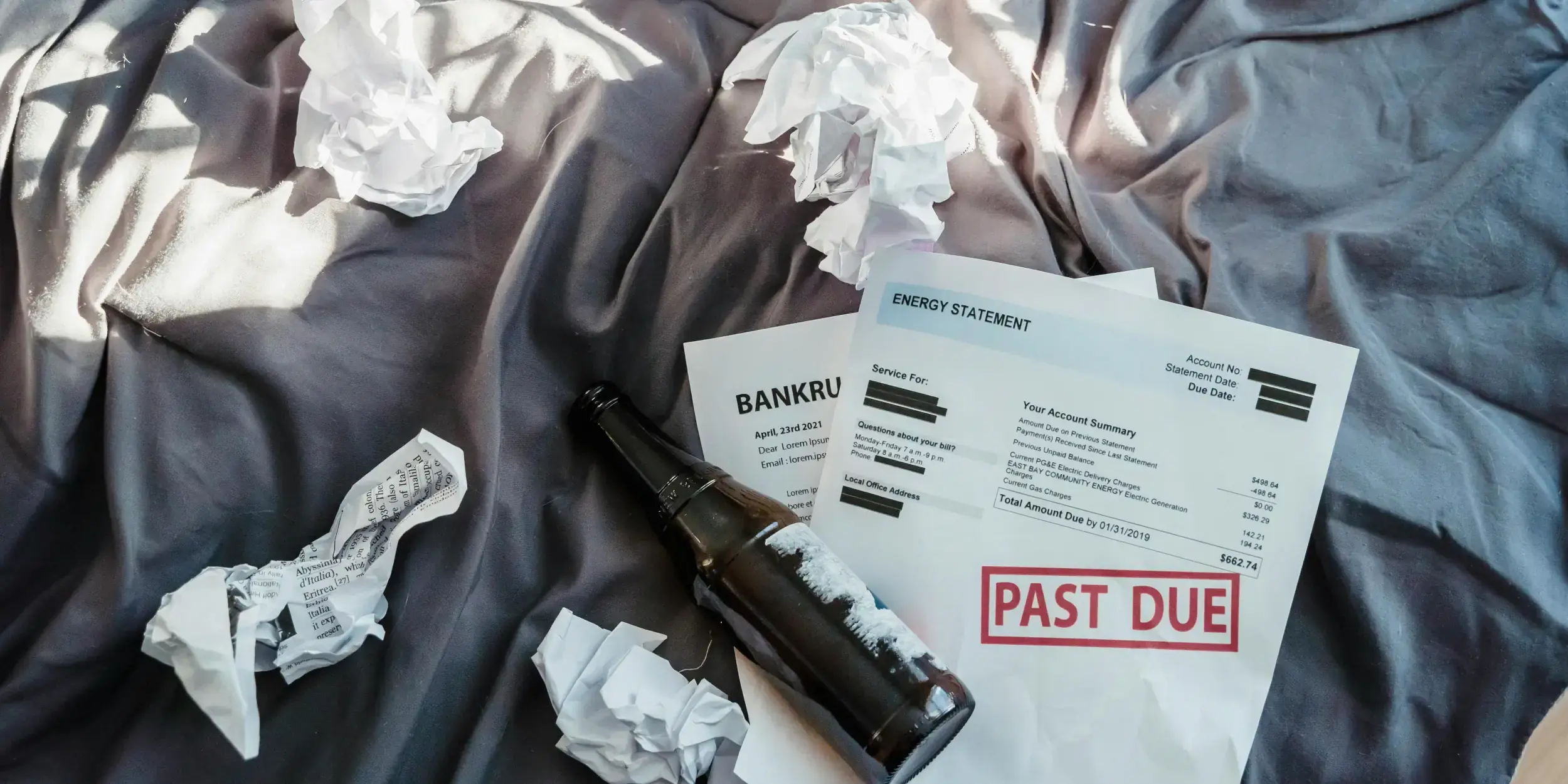
Is Hosting on Netlify Going to Bankrupt you?
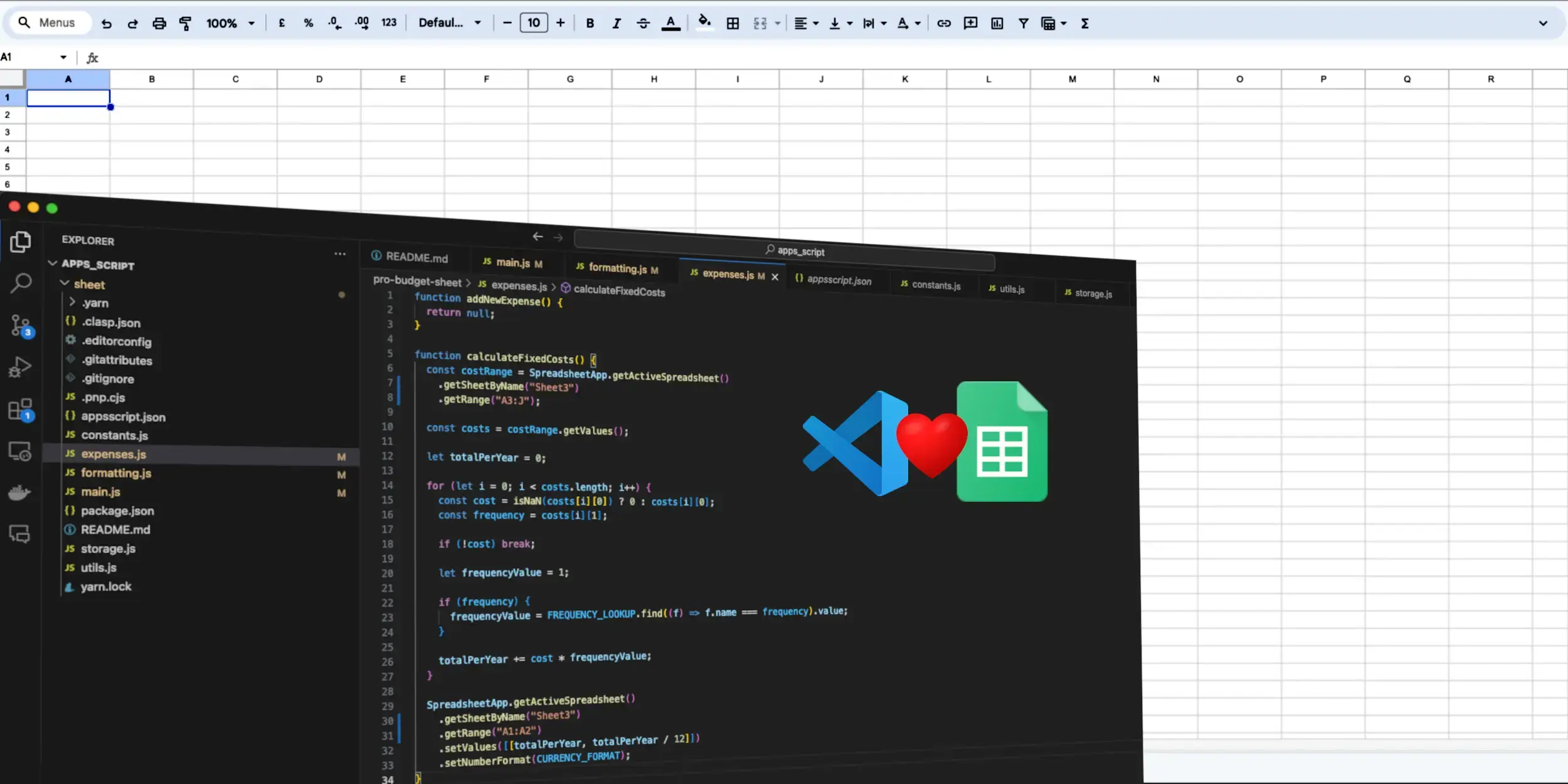
Develop Google Apps Script Code in Your Local Code Editor

Limit the Number of Pre-rendered Pages in Next.js

Understanding PostgreSQL RLS, and When to Use it

Build a Weather Widget Using Next.js
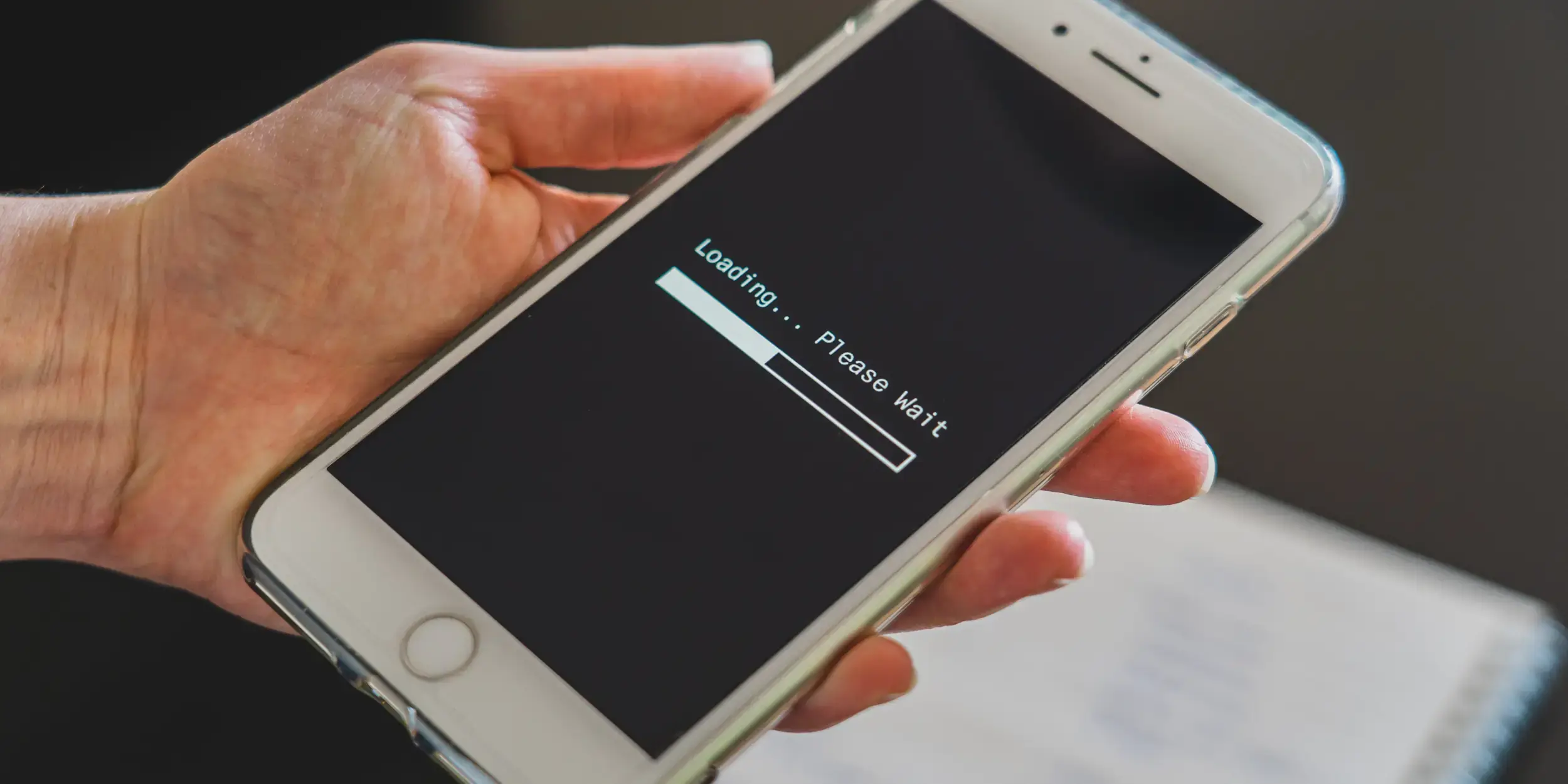
5 Ways to Redirect URLs in Next.JS
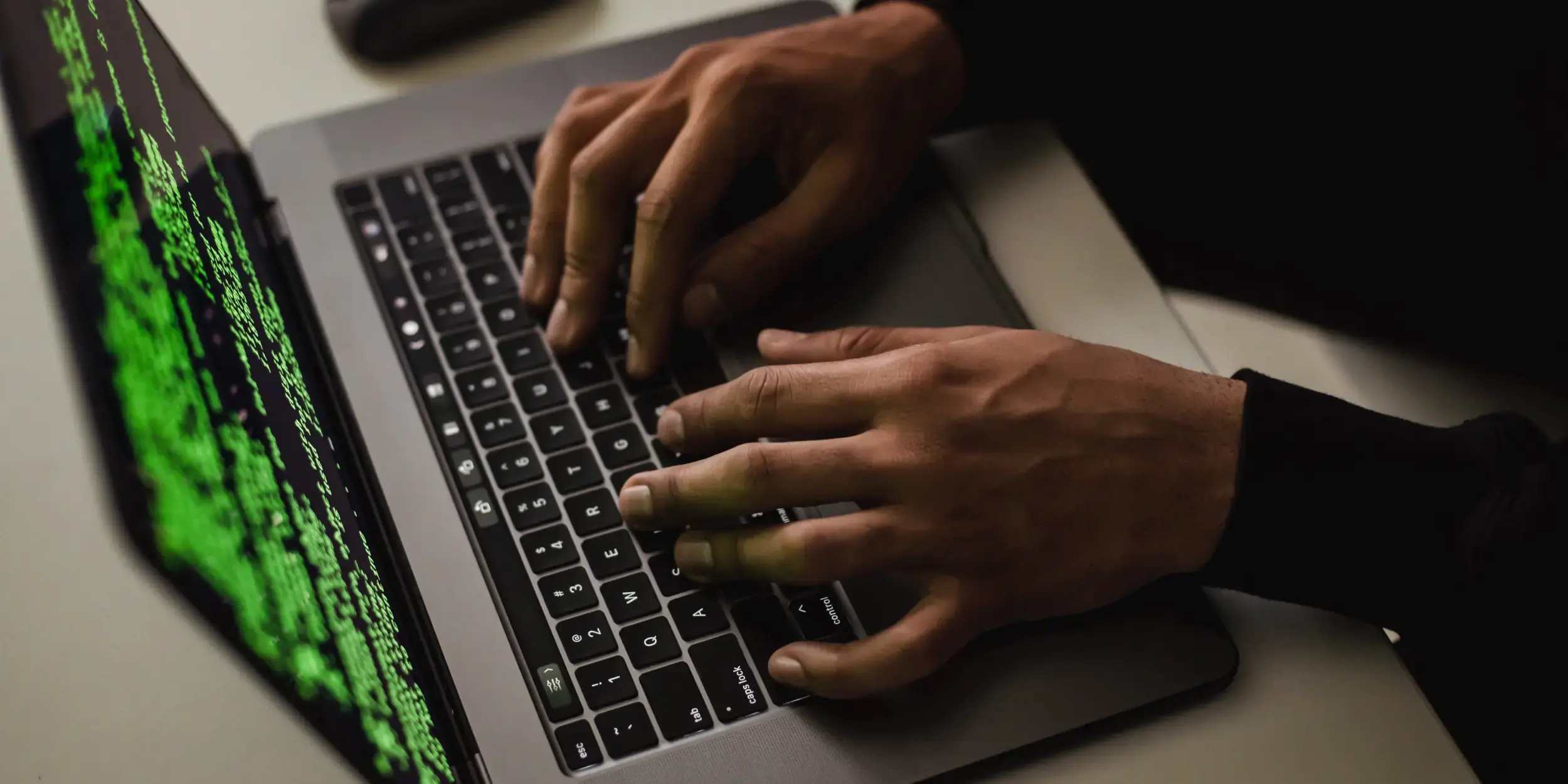
Fix Path Name Mismatches in Local & Remote Git Repos
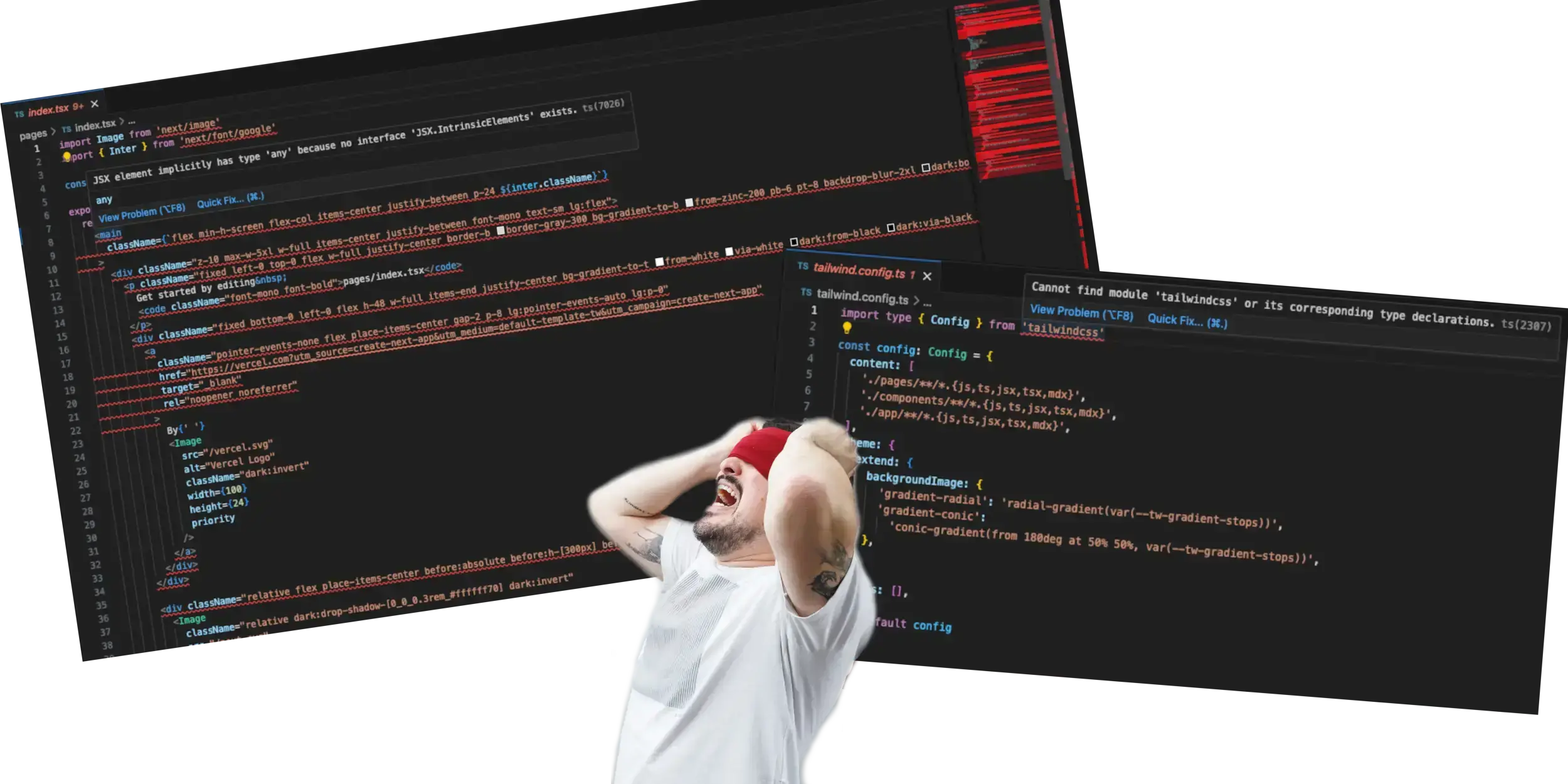
Fix "Cannot find module ..." TypeScript errors in VS Code

Fix "Unknown at rule @tailwind" errors in VS Code
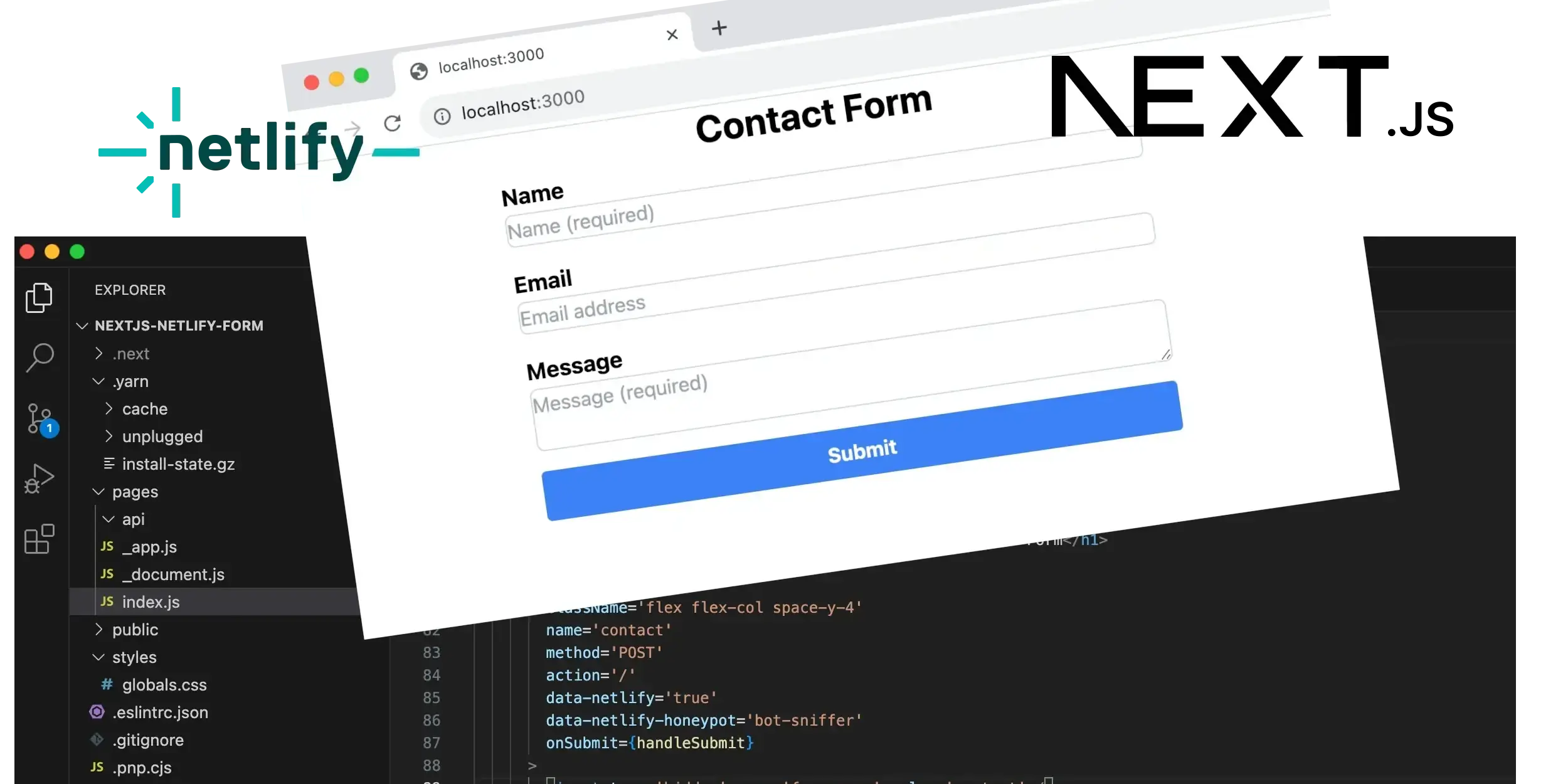
Build a Simple Contact Form with Next.JS and Netlify
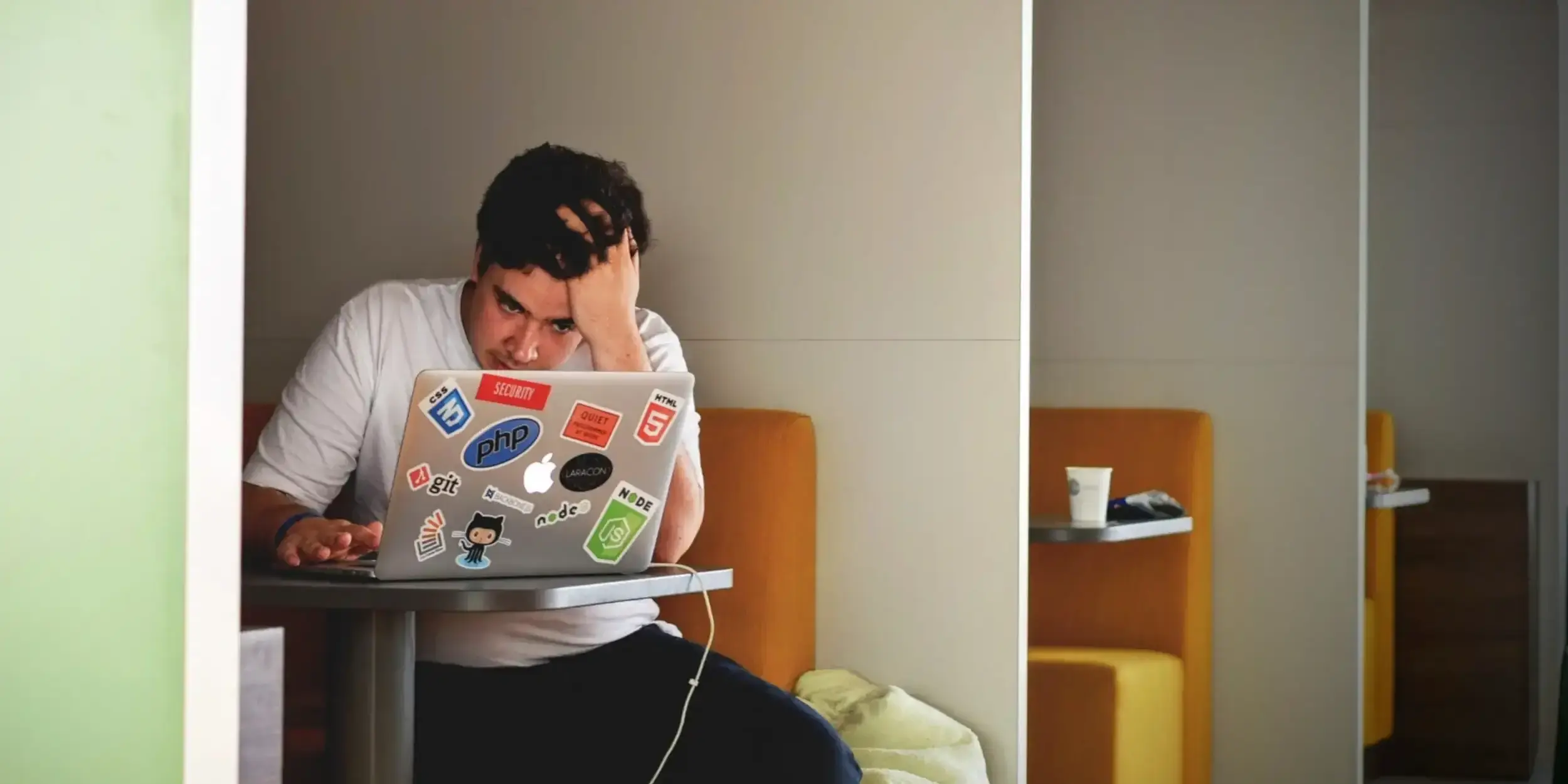
Fix "Hydration failed ..." Errors in React
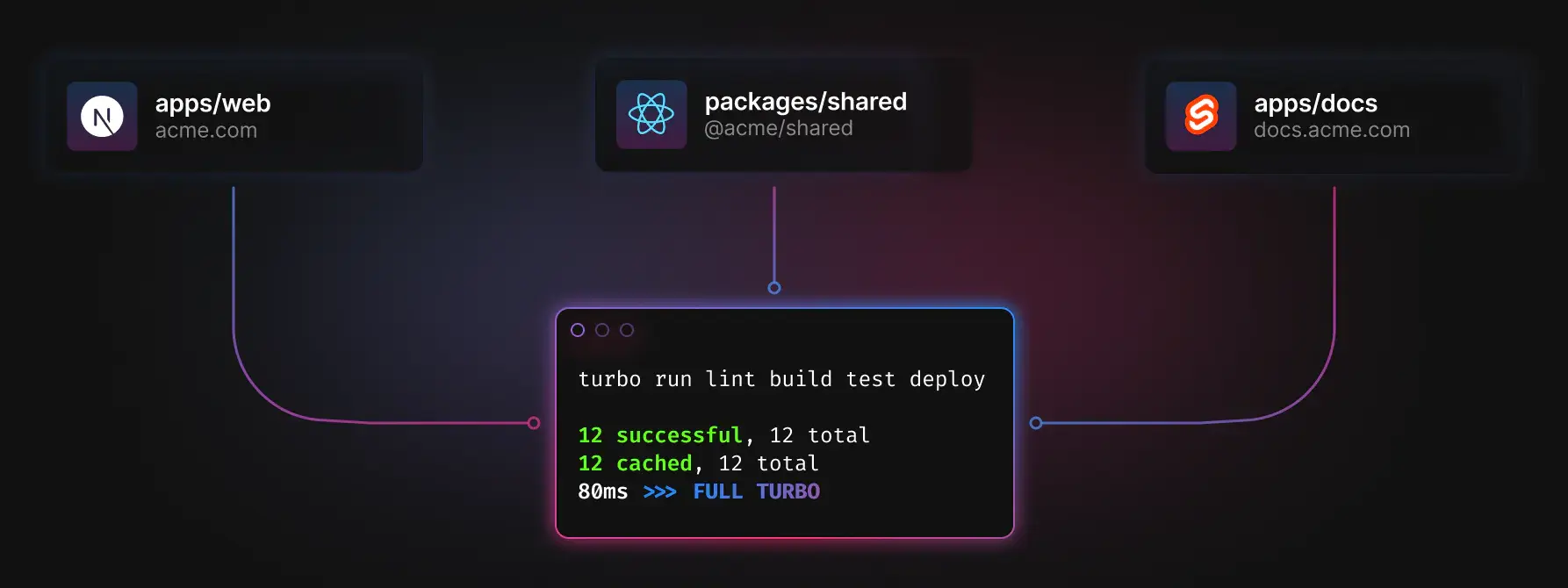
Updating Turborepo to use Yarn Berry

The Pros and Cons of Using a Monorepo
Comments